Archive
This post is archived and may contain outdated information. It has been set to 'noindex' and should stop showing up in search results.
This post is archived and may contain outdated information. It has been set to 'noindex' and should stop showing up in search results.
PHP: Prevent MySQL Code Injection Exploits
Apr 5, 2012ProgrammingComments (0)
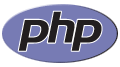
Any data that comes from the client-side needs to be properly validated and escaped before using. This is extra important if you're using that data to form part of a database query. Always assume that your users will try to input incorrect information and special characters into fields, and do not rely on HTML or JavaScript to prevent this.
Take a look at this simple, unprotected sign in script:
$q = mysql_query('SELECT * FROM users WHERE password = "' . $_POST['pass'] . '"');
if(mysql_num_rows($q) == 1)
{
// Signed in!
}
This can be easily exploited by the user inputting this into the password field (assuming they can guess the column name):
asdf" OR password LIKE "%%
What this does is insert an additional conditional check into the MySQL query (thanks to the unescaped quotes), allowing it to match any password. The actual MySQL query that PHP compiles and sends to the MySQL database will end up looking like this:
SELECT * FROM users WHERE password = "asdf" OR password LIKE "%%"
To avoid this exploit, you should use the mysql_real_escape_string function on the user input before inserting it into a database query. This function requires an open database connection and escapes any exploitable or error-causing characters (\x00, \n, \r, \, ', " and \x1a):
$pass = mysql_real_escape_string($_POST['pass']);
$q = mysql_query('SELECT * FROM users WHERE password = "' . $pass . '"');
if(mysql_num_rows($q) == 1)
{
// Signed in!
}