Archive
This post is archived and may contain outdated information. It has been set to 'noindex' and should stop showing up in search results.
This post is archived and may contain outdated information. It has been set to 'noindex' and should stop showing up in search results.
PHP: Use Identical Operator When Comparing User Input (Equals vs Identical)
Apr 1, 2012ProgrammingComments (0)
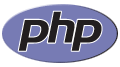
If you compare an integer to a string, PHP will evaluate the string as a 0 integer unless that string starts with a number, in which case it will truncate the non-number characters. This may be over-simplifying things a bit. Here are some examples:
var_dump('asdf' == 0); // true!
var_dump('asdf' == '0'); // false
var_dump('3sdf' == 0); // false
var_dump('3sdf' == 3); // true!
I'm sure you can imagine a scenario in which you compare user input against a string, and that user manages to insert a zero integer as input, resulting in a true result for the comparison. Something like this:
$user_input = 0;
$password = 'x83kKi77w';
if($user_input == $password)
{
$loggedin = true;
}
How to fix it? It's very easy; just use PHP's "identical" operator (===, that's three equals signs instead of two). What it does is check to make sure the two data values are of the same type as well as making sure they are equal to each other.
The above examples with an identical operator should now act as you expect:
var_dump('asdf' === 0); // false
var_dump('asdf' === '0'); // false
var_dump('3sdf' === 0); // false
var_dump('3sdf' === 3); // false